PHP Joomla: Generate certificates with the mpdf library
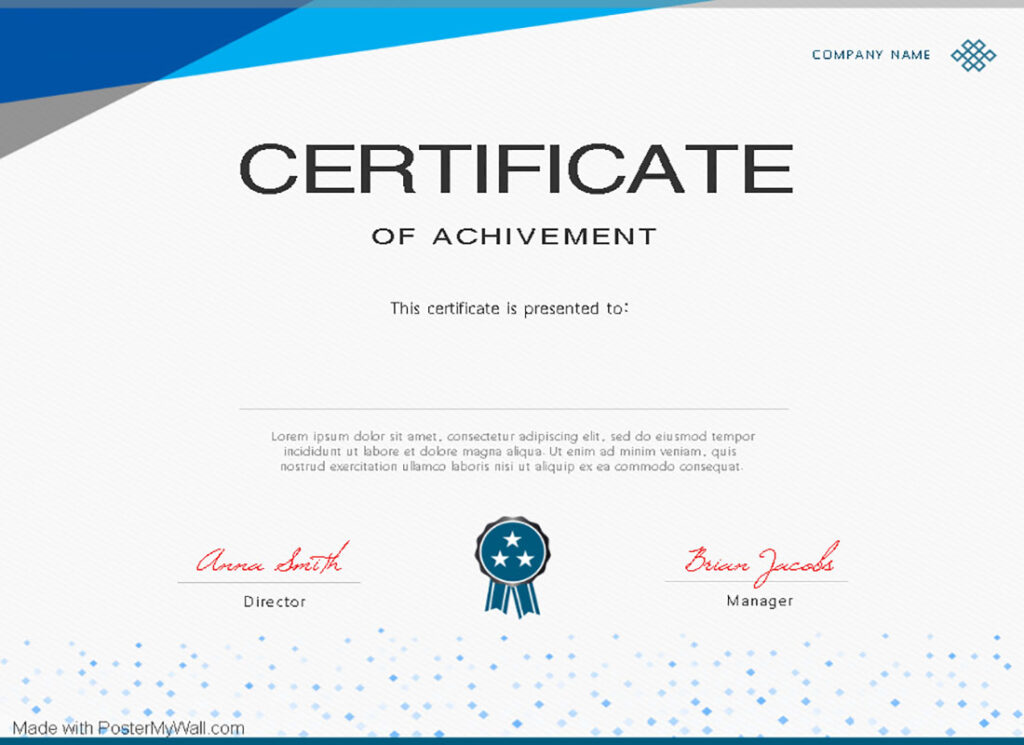
The company I work for happens to have to issue various certificates to many users, it would be very tiring if I had to input user names one by one.
By generating the certificate directly through programming, we can send the certificate directly to the user’s email, without having to print and share
Here is an example of a script that generates a certificate:
<?php
error_reporting(E_ALL);
define('_JEXEC', 1);
define('JPATH_BASE', dirname(__FILE__)); // should point to joomla root
define('DS', DIRECTORY_SEPARATOR);
defined('_JEXEC') or die();
require_once(JPATH_BASE . DS . 'includes' . DS . 'defines.php');
require_once(JPATH_BASE . DS . 'includes' . DS . 'framework.php');
/*To use Joomla's database classs*/
// gunakan perintah dibawah untuk joomla ver 3.6 kebawah
// disable jika versi di atas 3.6
require_once(JPATH_BASE . DS . 'libraries' . DS . 'joomla' . DS . 'factory.php');
// gunakan perintah dibawah untuk joomla ver 3.6 ke atas
// enable jika versi di atas 3.6
//require_once (JPATH_BASE.DS.'libraries'.DS.'joomla'.DS.'platform.php');
$mainframe = JFactory::getApplication('site');
$mainframe->initialise();
$user = JFactory::getUser(); // get user info
jimport('joomla.database.table');
jimport('joomla.database.table.table');
include("libraries/mpdf/mpdf.php");
$db = JFactory::getDBO();
$sql = "SELECT nama, jurusan, ipk
FROM mahasiswa ";
$db->setQuery($sql);
echo $sql;
$result = $db->query();
$num_rows = $db->getNumRows();
echo "Numrows : " . $num_rows;
// check result
if ($num_rows > 0) {
$result = $db->loadAssocList();
foreach ($result as $row) {
$mpdf = new mPDF('', 'A4', 0, 0, 0, 0, 0, 0);
$mpdf->setHeader(); // Clear headers before adding page
$mpdf->AddPage('L', 0, 0, 0, 0, 0, 0);
$mpdf->ignore_invalid_utf8 = true;
$name = $row["nama"];
$ipk = $row["ipk"];
$images = "template-image.jpg";
$newtext = wordwrap($name, 30, "<br>");
$html = '
<style>
body {
font-family: Arial;
background-image: url(' . JURI::base() . 'images/' . $images . ');
color:#1F497D;}
h1 {font-family: Arial;font-size:40px;}
h2 {font-family: Arial;font-size:24px;}
.myfixed {
position: absolute;
font-family: Arial;font-size:40px;
overflow: visible;
left: 0;
right: 0;
top: 53mm;
width: 250mm; /* you must specify a width */
margin-top: auto;
margin-bottom: auto;
margin-left: auto;
margin-right: auto;
font-weight:bold;
}
.bpmTopicC td, .bpmTopicC td p { text-align: center; }
.bpmTopicC {
width:96%;
/*background-color: #e3ece4;*/
position: absolute;
font-family: Arial;
font-size:40px;
overflow: visible;
left: 0;
right: 0;
top: 52;
width: 250mm; /* you must specify a width */
margin-top: auto;
margin-bottom: auto;
margin-left: auto;
margin-right: auto;
font-weight:bold;
}
</style></pre>
<div class="myfixed">
<table class="bpmTopicC">
<tbody>
<tr>
<td>' . $newtext . '</td>
</tr>
</tbody>
</table>
</div>';
$mpdf->WriteHTML($html);
$mpdf->WriteFixedPosHTML('<div style="font-weight: bold;">' . $ipk . "</div>", 64, 74, 50, 100, 'auto');
$filename_replaced = str_replace('/', '', $name);
$mpdf->Output("files/$filename_replaced.pdf", "F");
//$mpdf->Output("files/$name".".pdf","I");
}
} else {
JFactory::getApplication()->enqueueMessage('No record found for this course', 'error');
//header('Location: '.$_SERVER['REQUEST_URI']);
JError::raiseWarning(100, 'Warning');
//header('Location: ' . $_SERVER["HTTP_REFERER"] );
JError::raiseWarning(100, 'Warning');
}
Requirements:
1. Latest Joomla
2. The MPDF library is installed in the library folder
3. Student table