Easy script to make simple lookup with Colorbox popup
In making applications, we often need a window to search for certain data, this function we usually call lookup.
This time we will create a simple lookup script with the popup library colorbox for the web.
The following is a lookup script for the web that functions to display and select data from all existing data lists.
Display the results of the script
Steps:
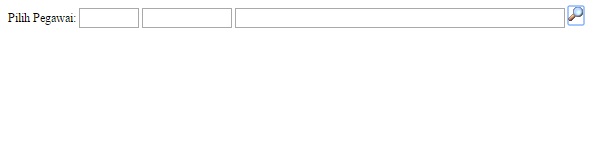
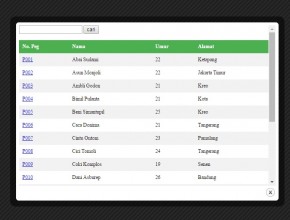
1. First create an employee table in the test database
CREATE TABLE `pegawai` ( `no_int` int(5) NOT NULL auto_increment, `NIP` char(5) collate latin1_general_ci default NULL, `nama` varchar(30) collate latin1_general_ci NOT NULL, `umur` int(2) NOT NULL, `alamat` varchar(100) collate latin1_general_ci NOT NULL, PRIMARY KEY (`no_int`) );
2. Create an index.html file with the following code:
<html>
<head>
<title>Searching By Ajax</title>
<link rel="stylesheet" href="css/colorbox.css" />
<script src="js/jquery.min.js"></script>
<script src="js/jquery.colorbox.js"></script>
<script>
$(document).ready(function(){
//Examples of how to assign the ColorBox event to elements
$(".ajax").colorbox({width:'650px',height:'450px'});
});
</script>
<!-- new -->
<script language="javascript">
var my_variable = new Array(); // for example
function passingToParent(){
arrayOfStrings = my_variable[0].split(',');
for (var i=1; i < my_variable.length; i++) {
$('#' + arrayOfStrings[i-1]).val(my_variable[i]);
}
// single form
//parent.$.fn.colorbox.close();
// framework form
jQuery.colorbox.close();;
}
</script>
<style>
body,table,input{
font-size:12px
}
</style>
</head>
<body>
<form action="" method="post">
Pilih Pegawai: <input type="text" id="no_int" name="no_int" size="4">
<input id="id" type="text" name="pegawai" size="4" />
<input type="text" id="nama_pegawai" name="nama_pegawai" size="20" readonly />
<!-- semua element anchor dengan class="thickbox" akan otomatis menampilkan dialog thickbox,
isi href bisa dimasukan setting thickbox berupa width dan height dari dialog thickbox -->
<a href="daftar_pegawai.php" class="ajax" onClick="my_variable[0]='no_int,id,nama_pegawai'">
<img src="button_search.png" border="0" /></a>
<small id="divAlertPegawai"></small>
</form>
</body>
</html>
Explanation of the script: first we include the jquery script and colorbox, then we create a function passingToParent to receive data passing from the lookup to the form, the id name of the data receiving component is set on the link
<a href=”employee_registration.php” class=”ajax” onClick=”my_variable[0]=’no_int,id,employee_name'”>
with the onClick attribute containing the input id my_variable[0]=’no_int,id,employee_name
3. Create the file register_pegawai.php with the following code:
Explanation: input component to perform a search, can receive Enter as search submit, the script function findRecord will perform an ajax call to cari_pegawai.php with the search value from the search id, namely the input component, the result of the response is directly written to the result id 4. Create the file cari_pegawai.php with the following code: <script language="javascript">
function getSelected(no_int, id, nama){
var args = new Array();
for (var i = 0; i < arguments.length; i++)
window.parent.my_variable[i+1] = arguments[i];
window.parent.passingToParent();
}
</script>
<style>
table {
border-collapse: collapse;
width: 100%;
}
th, td {
text-align: left;
padding: 8px;
}
tr:nth-child(even){background-color: #f2f2f2}
th {
background-color: #4CAF50;
color: white;
}
</style>
<?php
if (empty($_POST["cari"]))
$_POST["cari"] = "";
$mysqli = new mysqli("localhost", "admin", "", "test");
$ssql = "SELECT * FROM pegawai
WHERE nip like '%$_POST[cari]%'
or nama like '%$_POST[cari]%'";
$query = $mysqli->query($ssql);
?>
<table bgcolor="#000000" cellspacing="1" cellpadding="3">
<tr bgcolor="#DDDDDD">
<th>No. Peg</th>
<th>Nama</th>
<th>Umur</th>
<th>Alamat</th>
</tr>
<?php while($row = $query->fetch_object()): ?>
<tr bgcolor="#FFFFFF">
<!-- fungsi selectPegawai di deklarasikan di index.html dan file ini bisa memanggilnya selama file ini
dipanggil oleh thickbox dari index.html, fungsi dari selectPegawai adalah untuk memasukan nilai
NIP dan nama pegawai dari masing-masing baris di daftar pegawai ini -->
<td align="center">
<a href="javascript:getSelected('<?=$row->no_int?>','<?=$row->NIP?>','<?=$row->nama?>')">
<?=$row->NIP?>
</a>
</td>
<td><?=$row->nama?></td>
<td align="center"><?=$row->umur?></td>
<td><?=$row->alamat?></td>
</tr>
<?php endwhile; ?>
</table>
Here’s a download link for a simple lookup sample file with a popup colorbox.
Notes:
The article above assumes that you already have knowledge about using mysql databases starting from login connections, creating databases, running scripts and creating tables
Gak bisa didownload gan sample-nya
cb lg gan sudah diupload
setelah klik cari, kenapa data pegawai tidak muncul ?
Keren, sederhana dan mudah dimengerti. Terima Kasih